In 2016, a group of Dutch hackers got their hands on Donald Trump’s credentials from the LinkedIn data breach. The data breach had happened a few years earlier in 2012 (news link here). His password? “yourfired” Curious to see if the password was reused, they attempted to log into Trump’s Twitter account and eventually got access to it. Using the same password that hadn’t been changed since before 2012 (link to read more about it here)
Even the future president at the time was in severe need of a better solution for generating unique and secure passwords.
Creating a Secure password generator from scratch with python
In a previous post we went over creating a password checker to check the security of your passwords but instead of brute forcing a secure password by hand trying to figure out the perfect password for you we are going to automate it by using python to make sure that every password we use is unique and secure
This Python script we’ll be writting today is designed to create secure, random passwords that meet standard security recommendations. The generator ensures each password includes a mix of lowercase and uppercase letters, digits, and symbols, avoiding predictable character patterns. The function generate_password
can be customized to generate passwords of any specified length, but defaults to 12 characters if no length is provided.
We’ll get started in our text / code editor of choice ill be using nano
Imports:
import random
import string
The script imports the random
module for generating random selections and the string
module, which provides a collection of string constants like uppercase letters, lowercase letters, digits, and punctuation.
Function Definition:
def generate_password(length=12):
This line defines a function named generate_password
with an optional parameter length
that defaults to 12. This parameter determines the length of the password generated.
Character Sets:
lowercase = string.ascii_lowercase
uppercase = string.ascii_uppercase
digits = string.digits
symbols = string.punctuation
The function sets up four strings containing different sets of characters: lowercase letters, uppercase letters, digits, and symbols (punctuation). These are retrieved from the string
module.
Combining Characters:
all_characters = lowercase + uppercase + digits + symbols
All the individual character sets are concatenated into one large string, all_characters
, which includes every possible character that could appear in the password.
Ensure Diversity:
password = random.choice(lowercase) + random.choice(uppercase) + random.choice(digits) + random.choice(symbols)
The script ensures that the password contains at least one lowercase letter, one uppercase letter, one digit, and one symbol. This is done by randomly selecting one character from each category and concatenating them.
Fill the Remaining Length:
password += ''.join(random.choice(all_characters) for _ in range(length - 4))
After the first four characters are set, the remaining part of the password (length – 4 characters) is filled by randomly selecting from the all_characters
pool. This is accomplished using a list comprehension inside a ''.join()
method, which efficiently constructs a string.
Shuffle for Randomness:
password = ''.join(random.sample(password, len(password)))
Even though the characters are selected randomly, the first four characters (one from each set) are always in the same sequence (lowercase, uppercase, digit, symbol). To ensure that these characters are not predictably placed at the start of the password, the script shuffles the entire password string. This is done by using random.sample()
to randomly reorder all the characters in the password string.
Return the Password:
return password
Finally, the function returns the shuffled password string.
Example Usage:
print(generate_password(12)) # You can change the length by passing a different number
This line demonstrates how to use the function, generating a 12-character password by default. You can pass a different value to generate a password of another length.
Putting it together and testing it against our password strength checker
Putting all the previously documented code.
import random
import string
def generate_password(length=12):
# Characters to include in the password
lowercase = string.ascii_lowercase
uppercase = string.ascii_uppercase
digits = string.digits
symbols = string.punctuation
all_characters = lowercase + uppercase + digits + symbols
password = random.choice(lowercase) + random.choice(uppercase) + random.choice(digits) + random.choice(symbols)
password += ''.join(random.choice(all_characters) for _ in range(length - 4))
password = ''.join(random.sample(password, len(password)))
return password
print(generate_password(12)) # You can change the length by passing a different number
Now you would save the password generator ‘password_gen.py’ in my case but save it to whatever you would like
Using the password generator is simple
python3 password_gen.py
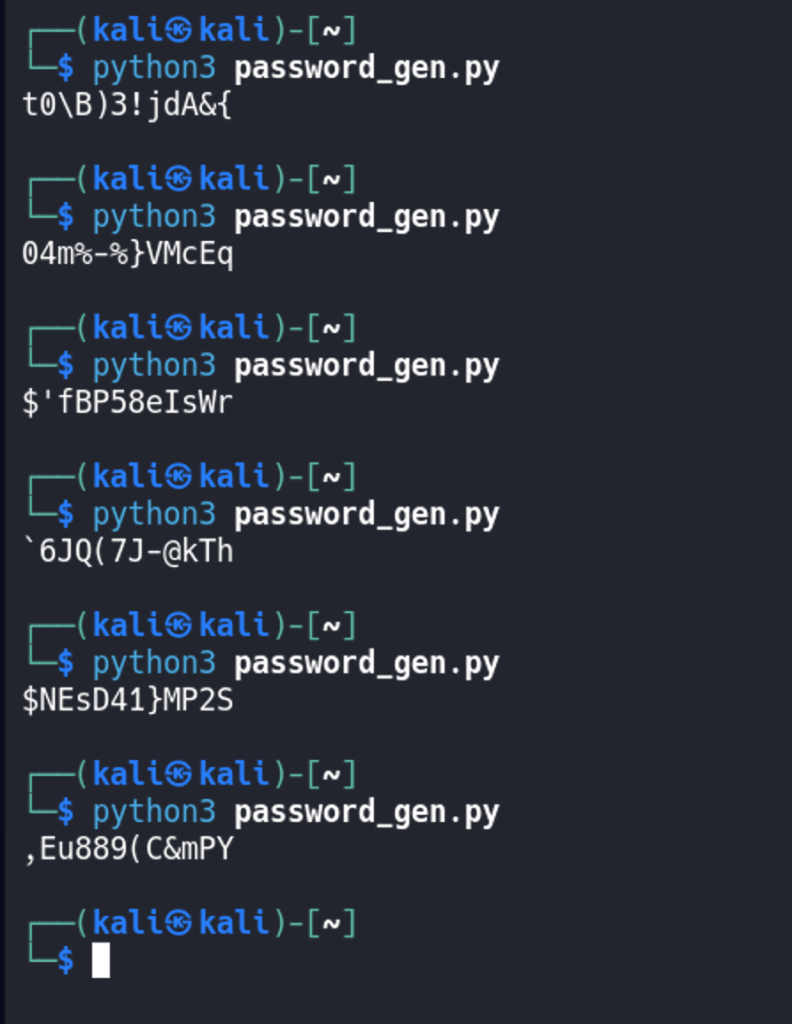
It gives us a unique and strong password everytime
Testing against our password checker
Running our password generator first and then using the output against our password checker
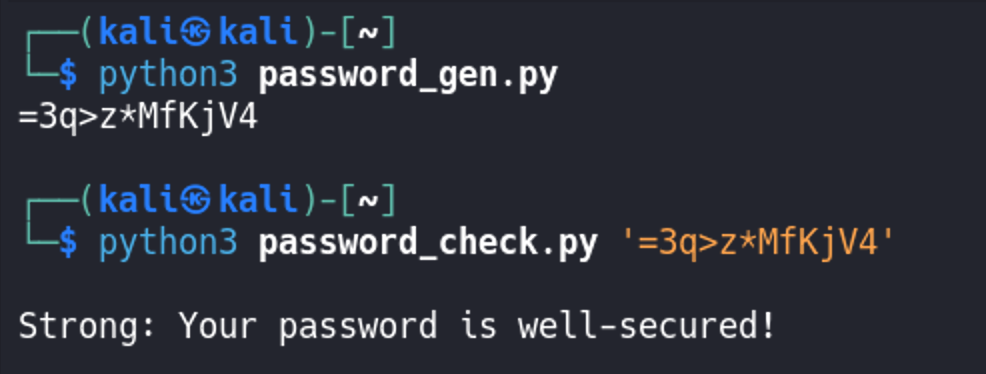
Thanks for reading through this guide on creating a password generator and learning about some real life examples of what not to do. Remember the importance of balancing security with simplicity. Keep experimenting, refining your tools, and strengthening your defenses. Stay sharp and secure—your digital safety depends on it.